Is this tool helpful?
How to Use the Code Analysis and Error Resolution Tool Effectively
This tool helps you quickly identify and fix coding errors by analyzing the specific code snippet you provide. Follow these simple steps to get the most accurate and useful results:
-
Enter the Code Causing the Issue:
Paste your problematic code snippet into the field labeled “The piece of code causing the error.” For example, you might enter:
def find_even_numbers(nums):
return [num for num in nums if num & 1 == 0]Or:
for i in range(5):
console.log(“Count: ” + i) -
Describe the Error You Encountered:
In the “Description of the error encountered” field, explain the issue clearly. Examples include:
The function returns an empty list instead of even numbers. No errors are shown but the output is incorrect.
Or:
The console shows “Count: undefined” instead of numbers 0 to 4.
-
Specify Your Programming Language:
Enter the programming language of your code, such as “Python” or “JavaScript”.
-
Provide Development Environment Details (Optional):
You can add information about your setup, for example, “Python 3.9.1, PyCharm” or “React 17, Chrome 90”. This helps tailor the analysis to your context.
-
Submit for Analysis:
Click the “Analyze Code and Provide Solution” button to send your information for processing.
-
Review the Analysis and Solutions:
Once processed, you’ll receive a detailed explanation of the error, possible causes, and step-by-step solutions.
-
Save or Share Results:
You can copy the analysis output to your clipboard for easy saving or sharing with your team.
What is the Code Analysis and Error Resolution Tool?
This tool is an AI-powered assistant designed to analyze your code snippets, diagnose errors, and suggest clear solutions. It helps developers like you spot syntax mistakes, logical bugs, and performance issues across many popular programming languages.
By interpreting your code and error descriptions, the tool acts like a knowledgeable programming mentor, helping reduce your debugging time and improving your code quality.
Core Benefits for Developers
- Faster Debugging: Quickly pinpoint root causes behind your errors.
- Clear Explanations: Understand why errors occur with step-by-step walkthroughs.
- Actionable Fixes: Receive practical solutions that you can implement immediately.
- Code Optimization: Get tips to improve speed, readability, and maintainability.
- Broad Language Support: Works with JavaScript, Python, Java, and many more programming languages.
- Best Practices Guidance: Encourages adherence to standard coding conventions.
Practical Use Cases for the Code Analysis and Error Resolution Tool
1. Fixing Syntax Errors Quickly
Suppose you have this Java snippet:
int total = 0
for(int i = 0; i < 10; i++) {
total += i;
// Missing closing bracket here
The tool will point out the missing closing bracket and show you the corrected structure:
int total = 0;
for(int i = 0; i < 10; i++) {
total += i;
}
2. Identifying Logical Errors
Consider this Python function to find the average of a list:
def average(numbers):
return sum(numbers) / len(sum(numbers))
The tool detects the misuse of len(sum(numbers)) instead of len(numbers) and provides an explanation plus the correct code:
def average(numbers):
return sum(numbers) / len(numbers)
3. Improving Code Performance
Here’s an example where you loop over an array multiple times:
let evens = [];
for(let i = 0; i < arr.length; i++) {
if(arr[i] % 2 === 0) evens.push(arr[i]);
}
let sum = 0;
for(let n of evens) sum += n;
The tool might recommend using a single pass with array methods for better efficiency:
const sum = arr.filter(n => n % 2 === 0).reduce((a,b) => a + b, 0);
4. Assisting in Code Reviews
When reviewing code, you can run complex snippets through this tool to highlight hidden bugs or suggest improvements before merging changes. It offers an objective second opinion that complements peer reviews.
5. Learning and Skill Development
If you’re learning a new programming language or framework, the tool clarifies errors and coding patterns, accelerating your progress with practical explanations that go beyond just fixing bugs.
Frequently Asked Questions About the Code Analysis and Error Resolution Tool
Can the tool analyze large projects with multiple files?
While best suited for individual code snippets, you can submit relevant sections from different files separately with a detailed description to get helpful insights on larger issues.
Does the tool support various coding styles?
Yes, it understands many coding conventions and adjusts recommendations to respect idiomatic usage in your chosen programming language.
Can it help optimize code for specific hardware or environments?
Yes, by providing details about your development environment, you receive tailored suggestions that improve performance for your setup.
Is the tool beginner-friendly?
Absolutely. It offers clear explanations and teaching moments that make it easy for those new to coding to learn and correct mistakes.
How regularly is the tool’s knowledge updated?
Its database updates constantly to keep pace with language advancements, new frameworks, and evolving best practices in software development.
Can I use this tool to improve documentation and code readability?
Yes, it often recommends clearer comments and coding conventions alongside fixes, helping you produce cleaner, more maintainable code.
Is the tool useful for collaborative coding?
While it doesn’t have built-in collaboration features, you can easily share analysis results with teammates by copying the output for discussion or code review.
Summary
This Code Analysis and Error Resolution Tool offers a straightforward way to analyze your code snippets, identify errors, and receive actionable solutions. You’ll save time debugging, improve the quality and performance of your code, and learn from each analysis. Whether you’re fixing a simple typo or untangling complex logic bugs, this tool fits smoothly into your development workflow, supporting you every step of the way.
Important Disclaimer
The calculations, results, and content provided by our tools are not guaranteed to be accurate, complete, or reliable. Users are responsible for verifying and interpreting the results. Our content and tools may contain errors, biases, or inconsistencies. We reserve the right to save inputs and outputs from our tools for the purposes of error debugging, bias identification, and performance improvement. External companies providing AI models used in our tools may also save and process data in accordance with their own policies. By using our tools, you consent to this data collection and processing. We reserve the right to limit the usage of our tools based on current usability factors. By using our tools, you acknowledge that you have read, understood, and agreed to this disclaimer. You accept the inherent risks and limitations associated with the use of our tools and services.
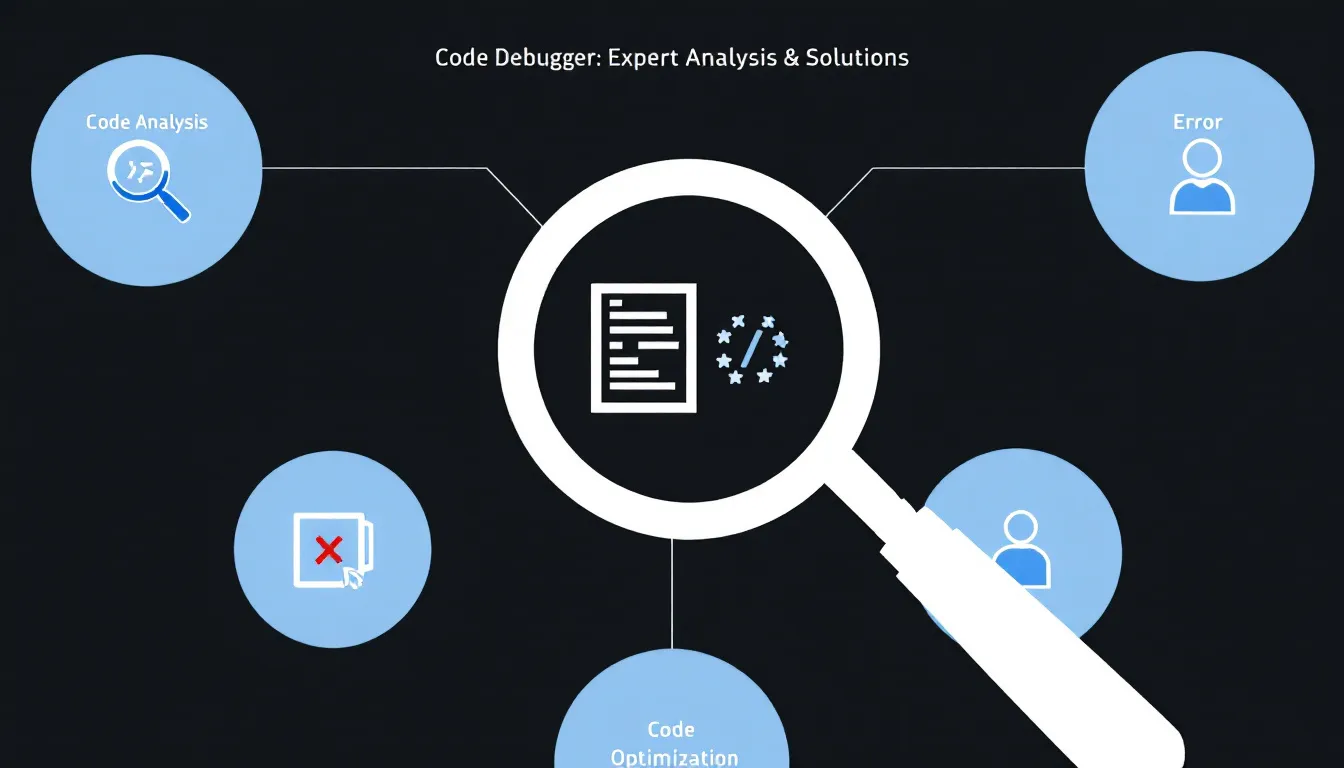