Code Explainer
Is this tool helpful?
How to Use the Code Explanation Tool Effectively
Our Code Explanation Tool is designed to provide detailed insights into any piece of code you’re working with or trying to understand. Here’s a step-by-step guide on how to use this tool effectively:
- Access the Tool: Navigate to the Code Explanation Tool on our website. You’ll see a clean, user-friendly interface with a text area for input.
- Paste Your Code: In the large text area labeled “Paste the code snippet you want explained,” copy and paste the code you want to be explained. For example, you might input:
function calculateFactorial(n) { if (n === 0 || n === 1) { return 1; } return n * calculateFactorial(n – 1); }
- Submit the Code: Once you’ve pasted your code, click the blue “Explain Code” button at the bottom of the form.
- Wait for Processing: The tool will process your code using advanced AI algorithms. This usually takes a few seconds, during which the button will display “Processing…”
- Review the Explanation: Once processing is complete, a detailed explanation will appear below the input form. This explanation will cover various aspects of your code, including its functionality, logic, and potential use cases.
- Copy the Explanation: If you want to save or share the explanation, use the “Copy to Clipboard” button at the bottom of the result section.
Remember, the more complex your code snippet, the more detailed the explanation will be. Don’t hesitate to use this tool for anything from simple functions to more intricate algorithms!
Unveiling the Power of Code: Your Personal Programming Mentor
In the vast and ever-evolving world of programming, understanding complex code can often feel like deciphering an ancient language. Whether you’re a novice programmer taking your first steps into the world of coding or a seasoned developer tackling a new challenge, our Code Explanation Tool serves as your personal programming mentor, ready to demystify any piece of code you encounter.
This innovative tool harnesses the power of advanced artificial intelligence to provide comprehensive, in-depth explanations of code snippets. It’s designed to break down the intricacies of programming logic, elucidate complex algorithms, and offer insights that can enhance your coding skills and understanding.
The Purpose and Benefits of the Code Explanation Tool
The primary purpose of our Code Explanation Tool is to bridge the gap between code and comprehension. It aims to make programming more accessible, understandable, and less intimidating for learners while serving as a valuable resource for experienced developers. Here are some key benefits:
- Accelerated Learning: By providing detailed explanations of code functionality, the tool helps users learn new programming concepts and techniques faster.
- Enhanced Understanding: It breaks down complex code into digestible explanations, making it easier to grasp intricate programming logic.
- Time-Saving: Instead of spending hours trying to decipher challenging code, users can get instant, comprehensive explanations.
- Improved Code Quality: By understanding best practices and potential optimizations, users can write better, more efficient code.
- Cross-Language Insights: The tool can explain code from various programming languages, helping users transition between different technologies.
Unlocking the Benefits of the Code Explanation Tool
Our Code Explanation Tool offers a myriad of benefits that cater to a wide range of users, from coding beginners to experienced developers. Let’s delve deeper into how this tool can revolutionize your coding experience:
1. Accelerated Learning Curve
One of the most significant advantages of using our Code Explanation Tool is its ability to dramatically speed up the learning process. When you’re new to programming or learning a new language, understanding how different code constructs work can be challenging. Our tool breaks down complex code into easily digestible explanations, allowing you to grasp concepts more quickly and efficiently.
For instance, if you’re learning about recursion, you might input a recursive function like this:
function fibonacci(n) { if (n <= 1) return n; return fibonacci(n - 1) + fibonacci(n - 2); }
The tool would then provide a detailed explanation of how recursion works in this context, helping you understand the concept more deeply and quickly than you might through traditional learning methods.
2. Enhanced Code Comprehension
Even experienced developers sometimes encounter code that’s difficult to understand at first glance. Our tool acts as a code interpreter, breaking down complex algorithms and explaining the logic behind them. This enhanced comprehension can be particularly useful when:
- Working with legacy code that lacks proper documentation
- Collaborating on large projects with multiple contributors
- Trying to understand and implement complex algorithms
By providing clear explanations, the tool helps you grasp the underlying principles and intentions behind the code, leading to better overall understanding and ability to work with or modify the code.
3. Time-Saving Efficiency
In the fast-paced world of software development, time is a precious commodity. Our Code Explanation Tool can save you countless hours that would otherwise be spent poring over documentation or trying to decipher complex code structures. Instead of getting stuck on a particularly challenging piece of code, you can quickly get a comprehensive explanation and move forward with your project.
This efficiency is particularly valuable when:
- Onboarding new team members to a project
- Reviewing pull requests in a timely manner
- Debugging issues in unfamiliar parts of a codebase
4. Improvement in Code Quality
Understanding best practices and potential optimizations is crucial for writing high-quality code. Our tool not only explains what the code does but also highlights best practices demonstrated within the code and suggests possible improvements. This feature can help you:
- Identify and eliminate code smells
- Implement more efficient algorithms
- Adhere to coding standards and best practices
By consistently using the tool and learning from its explanations, you can significantly improve the quality of your own code over time.
5. Cross-Language Learning
In today’s polyglot programming environment, developers often need to work with multiple programming languages. Our Code Explanation Tool supports a wide range of languages, making it an invaluable resource for developers looking to expand their skill set or work on multi-language projects.
For example, if you’re a JavaScript developer trying to understand a Python script, you could input the Python code:
def quicksort(arr): if len(arr) <= 1: return arr pivot = arr[len(arr) // 2] left = [x for x in arr if x < pivot] middle = [x for x in arr if x == pivot] right = [x for x in arr if x > pivot] return quicksort(left) + middle + quicksort(right)
The tool would then provide an explanation that not only describes how the quicksort algorithm works but also highlights Python-specific features used in the implementation, helping you bridge the gap between languages.
Addressing User Needs and Solving Specific Problems
Our Code Explanation Tool is designed to address a variety of user needs and solve specific problems that programmers often encounter. Let’s explore some of these scenarios and how our tool can help:
1. Deciphering Complex Algorithms
Problem: You’ve encountered a complex algorithm in a codebase, and you’re struggling to understand how it works.
Solution: Input the algorithm into our Code Explanation Tool. The tool will break down the algorithm step-by-step, explaining its logic, time complexity, and potential use cases. This detailed explanation can help you understand not just what the algorithm does, but why it does it that way.
For example, let’s say you encounter this implementation of the Depth-First Search algorithm:
function dfs(graph, start, visited = new Set()) { console.log(start); visited.add(start); for (let neighbor of graph[start]) { if (!visited.has(neighbor)) { dfs(graph, neighbor, visited); } } }
Our tool would explain how this algorithm traverses a graph, the role of the ‘visited’ set in preventing infinite loops, and how recursion is used to explore all paths.
2. Understanding Unfamiliar Design Patterns
Problem: You’re working on a project that uses design patterns you’re not familiar with.
Solution: Paste the code implementing the design pattern into our tool. It will not only explain how the code works but also identify the design pattern being used, its benefits, and common use cases. This can help you quickly get up to speed with new architectural approaches.
For instance, if you input code implementing the Observer pattern:
class Subject { constructor() { this.observers = []; } addObserver(observer) { this.observers.push(observer); } removeObserver(observer) { const index = this.observers.indexOf(observer); if (index > -1) { this.observers.splice(index, 1); } } notifyObservers() { for (let observer of this.observers) { observer.update(); } } }
The tool would explain the concept of the Observer pattern, how it’s implemented in this code, and why it’s useful for creating loosely coupled designs.
3. Optimizing Inefficient Code
Problem: You’ve identified a performance bottleneck in your application, but you’re not sure how to optimize the code.
Solution: Input the inefficient code into our tool. It will not only explain what the code does but also suggest potential optimizations. This can include algorithmic improvements, better use of data structures, or language-specific optimizations.
For example, if you input this inefficient code for finding prime numbers:
function isPrime(n) { for (let i = 2; i < n; i++) { if (n % i === 0) return false; } return n > 1; }
The tool might suggest optimizations like checking only up to the square root of n, or using the Sieve of Eratosthenes for finding multiple primes efficiently.
4. Translating Between Programming Languages
Problem: You need to port a function from one programming language to another, but you’re not fluent in the target language.
Solution: Input the original function into our Code Explanation Tool. The detailed explanation of how the function works can serve as a guide for implementing it in another language. While it won’t provide a direct translation, understanding the logic and algorithm can make the porting process much easier.
For instance, if you input a Python list comprehension:
squared_evens = [x**2 for x in range(10) if x % 2 == 0]
The tool would explain how this creates a list of squared even numbers from 0 to 9. With this understanding, you could more easily implement the same logic in a language that doesn’t support list comprehensions, like JavaScript:
const squaredEvens = Array.from({length: 10}, (_, i) => i) .filter(x => x % 2 === 0) .map(x => x ** 2);
5. Learning New Language Features
Problem: A new version of your programming language has been released with features you’re not familiar with.
Solution: As you encounter code using these new features, input it into our Code Explanation Tool. The tool will provide detailed explanations of how these new features work, their benefits, and potential use cases. This can help you quickly adapt to language evolution and make the most of new capabilities.
For example, if you’re a JavaScript developer learning about the new optional chaining operator, you might input:
const name = user?.profile?.name;
The tool would explain how this operator safely accesses nested object properties, preventing errors if an intermediate property is null or undefined.
Practical Applications and Use Cases
Our Code Explanation Tool has a wide range of practical applications across various scenarios in software development. Let’s explore some real-world use cases where this tool can be particularly valuable:
1. Code Review and Collaboration
In team environments, code reviews are crucial for maintaining code quality and knowledge sharing. Our tool can significantly enhance this process:
- Reviewer’s Perspective: When reviewing a pull request, you can use the tool to quickly understand complex changes or new algorithms introduced by your colleagues. This can help you provide more insightful feedback and catch potential issues more effectively.
- Author’s Perspective: Before submitting code for review, you can use the tool to generate clear explanations of your implementation. You can then include these explanations in your pull request description, making it easier for your reviewers to understand your code.
For example, if you’re reviewing a pull request that introduces a new sorting algorithm, you could use the tool to understand the algorithm’s efficiency and compare it to existing solutions.
2. Debugging and Troubleshooting
When faced with bugs or unexpected behavior, our Code Explanation Tool can be a powerful ally:
- Input the problematic code section to get a detailed breakdown of its functionality.
- Use the explanation to identify potential edge cases or logical errors that might be causing the issue.
- Understand how different parts of the code interact, which can help in isolating the source of the problem.
For instance, if you’re debugging a recursive function that’s causing a stack overflow, the tool’s explanation might help you identify the base case that’s not being reached, leading to infinite recursion.
3. Documentation Generation
Good documentation is crucial for maintainable code, but it’s often overlooked due to time constraints. Our tool can help in creating comprehensive documentation:
- Generate initial documentation drafts by inputting key functions or classes into the tool.
- Use the tool’s explanations to ensure you haven’t missed any important details in your manual documentation.
- Create more user-friendly documentation by using the tool’s explanations as a basis for writing tutorials or guides.
For example, you could use the tool to generate a detailed explanation of a complex API endpoint, which you can then refine and include in your API documentation.
4. Onboarding New Team Members
When new developers join a project, they often need to quickly get up to speed with the existing codebase. Our Code Explanation Tool can accelerate this process:
- New team members can use the tool to understand key components of the codebase without constantly interrupting their colleagues.
- Senior developers can use the tool to generate explanations of core algorithms or architectural decisions, creating a knowledge base for new joiners.
For instance, you could create a “key concepts” document for new team members by using the tool to explain the most important or complex parts of your codebase.
5. Learning and Skill Development
For both self-taught programmers and those in formal education, our tool can be a valuable learning aid:
- Students can use it to get detailed explanations of example code from textbooks or online courses.
- Developers looking to improve their skills can use it to understand advanced coding techniques or design patterns they encounter in open-source projects.
For example, a student learning about dynamic programming could input various implementations of the Fibonacci sequence calculation, from naive recursion to memoization and bottom-up approaches, to understand the evolution of the solution and the benefits of each approach.
6. Code Refactoring and Optimization
When working on improving existing code, our tool can provide valuable insights:
- Use it to fully understand the current implementation before making changes.
- Input refactored code to ensure that the new version maintains the same functionality as the original.
- Identify potential optimizations that might not be immediately obvious.
For instance, if you’re refactoring a complex data processing pipeline, you could use the tool to ensure you understand all the edge cases handled by the current implementation before you begin restructuring the code.
Frequently Asked Questions
Q1: Can the Code Explanation Tool handle any programming language?
A1: Our tool is designed to work with a wide range of popular programming languages, including but not limited to Python, JavaScript, Java, C++, Ruby, and Go. It can provide insights into the general logic and structure of code in most languages, even if it doesn’t have specific knowledge of every language feature.
Q2: How detailed are the explanations provided by the tool?
A2: The tool provides comprehensive explanations that cover various aspects of the code, including its functionality, logic, potential use cases, and even suggestions for optimization. The level of detail scales with the complexity of the input code – simple snippets will receive concise explanations, while complex algorithms or design patterns will be explained in greater depth.
Q3: Can the tool explain entire programs or just small code snippets?
A3: While the tool can handle a wide range of input sizes, it’s most effective with focused code snippets rather than entire programs. For best results, we recommend inputting specific functions, classes, or algorithms that you want to understand in detail. If you need to understand a larger program, consider breaking it down into smaller, logical components and analyzing each separately.
Q4: Does the tool provide information about code performance or complexity?
A4: Yes, when relevant, the tool will provide insights into the time and space complexity of algorithms. It may also suggest potential optimizations or alternative approaches that could improve performance. However, for precise performance metrics, you should still rely on profiling tools specific to your programming environment.
Q5: How often is the tool updated with new programming languages or features?
A5: We strive to keep our Code Explanation Tool up-to-date with the latest developments in the programming world. The tool is regularly updated to include new language features and emerging programming paradigms. However, the exact frequency of updates may vary depending on the pace of changes in different programming languages.
Q6: Can the tool help me learn a new programming language?
A6: While the Code Explanation Tool is not a substitute for structured learning resources, it can be a valuable supplementary tool when learning a new language. By inputting example code in the new language, you can get detailed explanations that help you understand how different language features and syntax work in practice.
Q7: Is there a limit to how much code I can input at once?
A7: To ensure optimal performance and accuracy, we recommend keeping your input to manageable chunks of code – typically not more than a few hundred lines at a time. For larger codebases, it’s best to break them down into smaller, logically distinct components and analyze each separately.
Q8: Can the tool explain code that contains syntax errors?
A8: The tool works best with syntactically correct code. While it may be able to provide some insights into code with minor syntax errors, for the most accurate and helpful explanations, it’s best to ensure your input code is free of syntax errors before submission.
Q9: Does the tool provide explanations in languages other than English?
A9: Currently, our Code Explanation Tool provides explanations in English. We’re exploring the possibility of adding support for other languages in future updates to make the tool accessible to a wider global audience.
Q10: Can I use the explanations generated by this tool in my own projects or documentation?
A10: Yes, you’re welcome to use the explanations generated by our tool in your own projects, documentation, or learning materials. We recommend reviewing and potentially editing the output to ensure it fits perfectly with your specific context and needs.
Important Disclaimer
The calculations, results, and content provided by our tools are not guaranteed to be accurate, complete, or reliable. Users are responsible for verifying and interpreting the results. Our content and tools may contain errors, biases, or inconsistencies. We reserve the right to save inputs and outputs from our tools for the purposes of error debugging, bias identification, and performance improvement. External companies providing AI models used in our tools may also save and process data in accordance with their own policies. By using our tools, you consent to this data collection and processing. We reserve the right to limit the usage of our tools based on current usability factors. By using our tools, you acknowledge that you have read, understood, and agreed to this disclaimer. You accept the inherent risks and limitations associated with the use of our tools and services.
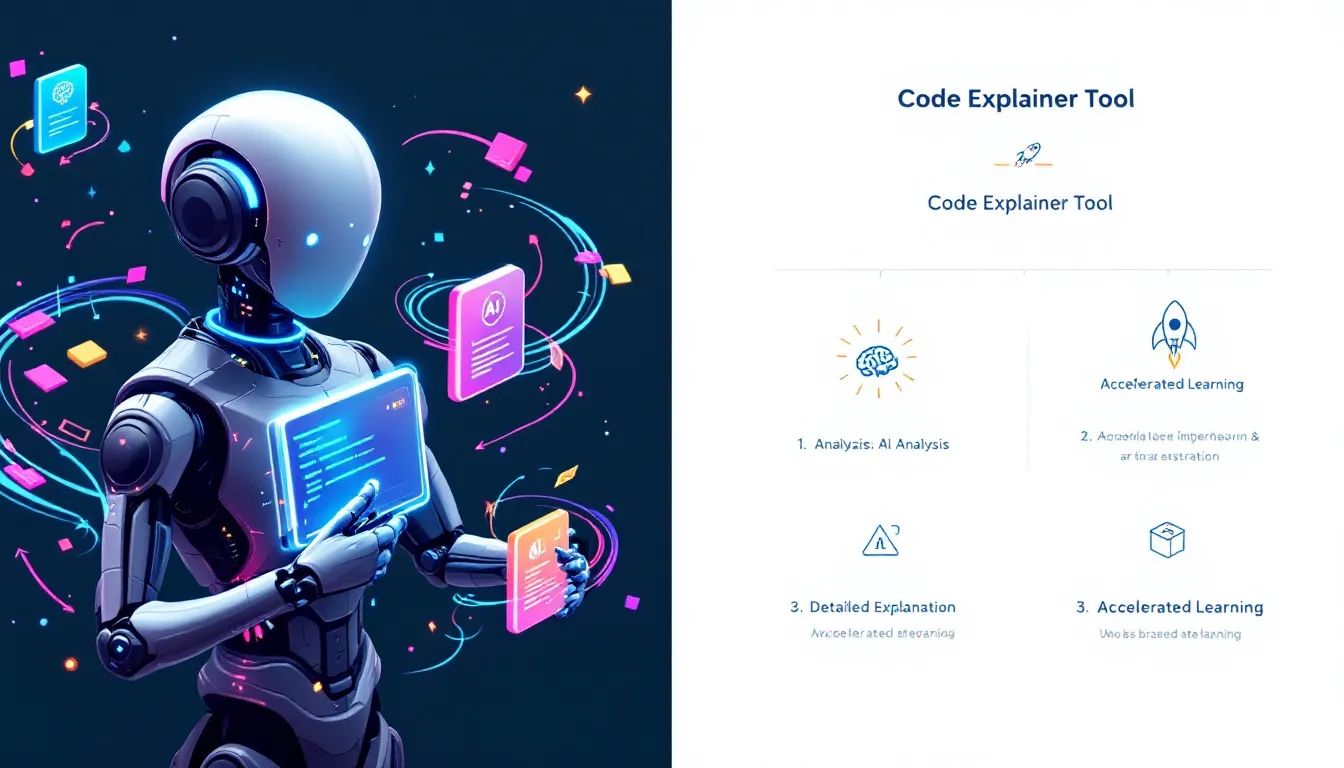