Code Refactoring Tool
Is this tool helpful?
How to Use the Code Refactoring Tool Effectively
Our Code Refactoring Tool is designed to help developers improve their code quality and efficiency. Here’s a step-by-step guide on how to use this tool effectively:
- Paste Your Code Snippet: In the first text area labeled “The piece of code to be refactored,” paste the code snippet you want to refactor. For example, you might input a complex function like this:function calculateFactorial(n) { if (n === 0 || n === 1) { return 1; } else { return n * calculateFactorial(n – 1); } }
- Specify the Programming Language: In the input field labeled “The programming language of the code snippet,” enter the language of your code. For instance, you might enter “JavaScript” for the above example.
- Add Specific Concerns (Optional): If you have any particular areas you want the tool to focus on during refactoring, enter them in the “Any specific concerns or areas to focus on during refactoring” text area. For example, you might write: “Improve performance and readability, consider using iterative approach instead of recursion.”
- Submit for Refactoring: Click the “Refactor Code” button to submit your code for analysis and refactoring.
- Review the Results: Once the refactoring process is complete, the tool will display the refactored code in the “Refactored Code” section below the form.
- Copy the Refactored Code: If you’re satisfied with the refactored version, click the “Copy to Clipboard” button to easily transfer the improved code to your development environment.
Introduction to Code Refactoring
Code refactoring is the process of restructuring existing computer code without changing its external behavior. It’s a crucial practice in software development that aims to improve the non-functional attributes of the software. Our Code Refactoring Tool leverages advanced algorithms and best practices to enhance your code’s efficiency, readability, and maintainability.
What is Code Refactoring?
Code refactoring involves modifying the internal structure of code without altering its external functionality. It’s like renovating a house: you’re improving its structure and efficiency without changing its outward appearance. The main goals of refactoring include:
- Improving code readability
- Reducing complexity
- Enhancing maintainability
- Increasing extensibility
- Optimizing performance
The Importance of Code Refactoring
In the fast-paced world of software development, code refactoring is not just a luxury—it’s a necessity. As codebases grow and evolve, they can become complex and difficult to maintain. Regular refactoring helps keep code clean, efficient, and adaptable to future changes. It’s an investment in the long-term health and sustainability of your software projects.
Benefits of Using the Code Refactoring Tool
Our Code Refactoring Tool offers numerous advantages for developers of all skill levels. Here are some key benefits:
1. Time Savings
Manual refactoring can be a time-consuming process, especially for large codebases. Our tool automates many aspects of refactoring, significantly reducing the time required to improve your code. This allows developers to focus on more creative and strategic aspects of their projects.
2. Consistency in Code Quality
The tool applies consistent refactoring patterns and best practices across your codebase. This ensures a uniform level of code quality, which is particularly valuable in team environments where multiple developers contribute to the same project.
3. Learning Opportunity
For less experienced developers, the tool serves as an educational resource. By analyzing the refactored code, developers can learn about efficient coding practices, design patterns, and language-specific optimizations.
4. Performance Optimization
The tool identifies and resolves performance bottlenecks in your code. This can lead to faster execution times and more efficient resource utilization, which is crucial for scalable applications.
5. Enhanced Code Maintainability
By simplifying complex logic and improving code structure, the tool makes your codebase easier to maintain and update. This is particularly beneficial for long-term projects or when onboarding new team members.
6. Bug Detection and Prevention
While the primary goal is not bug fixing, the refactoring process often reveals hidden bugs or potential issues in the code. By simplifying and clarifying the code structure, it becomes easier to spot and prevent future bugs.
Addressing User Needs and Solving Specific Problems
Our Code Refactoring Tool is designed to address a variety of common challenges faced by developers. Let’s explore how it tackles specific problems:
Reducing Code Complexity
Complex code is often difficult to understand and maintain. Our tool simplifies overly complicated code structures. For example, consider this nested if-else statement:
if (condition1) { if (condition2) { if (condition3) { // Do something } else { // Do something else } } else { // Another action } } else { // Default action }
The tool might refactor this into a more readable structure using early returns or switch statements:
if (!condition1) { // Default action return; }if (!condition2) { // Another action return; }if (condition3) { // Do something } else { // Do something else }
Optimizing Performance
The tool identifies performance bottlenecks and suggests optimizations. For instance, it might transform a recursive function into an iterative one to improve efficiency:
Original recursive function:
function fibonacci(n) { if (n <= 1) return n; return fibonacci(n - 1) + fibonacci(n - 2); }
Refactored iterative version:
function fibonacci(n) { if (n <= 1) return n; let a = 0, b = 1; for (let i = 2; i <= n; i++) { let c = a + b; a = b; b = c; } return b; }
Improving Code Readability
The tool enhances code readability by suggesting better variable names, breaking down long functions, and applying consistent formatting. For example:
Original code:
function calc(a, b, c) { return a * b + c; }
Refactored code:
function calculateTotal(quantity, unitPrice, taxAmount) { const subtotal = quantity * unitPrice; return subtotal + taxAmount; }
Applying Design Patterns
The tool recognizes opportunities to apply design patterns that can improve code structure and flexibility. For instance, it might suggest refactoring code to use the Factory pattern:
Original code:
class Car { constructor(make, model) { this.make = make; this.model = model; } }class Truck { constructor(make, model, payload) { this.make = make; this.model = model; this.payload = payload; } }function createVehicle(type, make, model, payload) { if (type === ‘car’) return new Car(make, model); if (type === ‘truck’) return new Truck(make, model, payload); }
Refactored code using Factory pattern:
class VehicleFactory { createVehicle(type, make, model, payload) { if (type === ‘car’) return new Car(make, model); if (type === ‘truck’) return new Truck(make, model, payload); throw new Error(‘Invalid vehicle type’); } }const factory = new VehicleFactory(); const car = factory.createVehicle(‘car’, ‘Toyota’, ‘Corolla’); const truck = factory.createVehicle(‘truck’, ‘Ford’, ‘F-150’, 2000);
Practical Applications and Use Cases
Our Code Refactoring Tool has a wide range of practical applications across various development scenarios. Here are some real-world use cases:
1. Legacy Code Modernization
Many organizations have legacy codebases that are difficult to maintain and extend. Our tool can help modernize such code, making it more compatible with current development practices and easier to integrate with newer systems.
Example: A financial institution with a 15-year-old trading system written in C++ could use the tool to refactor critical parts of the codebase. This might involve breaking down monolithic functions, introducing modern C++ features, and improving error handling mechanisms.
2. Preparing for Code Reviews
Developers can use the tool to polish their code before submitting it for review. This can lead to more productive code reviews, focusing on architectural and strategic aspects rather than basic code quality issues.
Example: A junior developer working on a new feature for a web application can run their JavaScript code through the tool before submitting a pull request. The tool might suggest using arrow functions, applying destructuring, or utilizing more efficient array methods, thereby improving the code quality before peer review.
3. Continuous Integration/Continuous Deployment (CI/CD) Pipeline Integration
The refactoring tool can be integrated into CI/CD pipelines to automatically improve code quality as part of the build and deployment process.
Example: In a microservices architecture, the tool could be set up to automatically refactor and optimize each service’s code during the build process. This ensures that all deployed code meets a minimum standard of quality and efficiency.
4. Technical Debt Reduction
Over time, quick fixes and rushed implementations can lead to technical debt. Our tool can help systematically reduce this debt by improving code structure and efficiency.
Example: An e-commerce platform that has grown rapidly might have accumulated technical debt in its order processing system. The refactoring tool could be used to streamline complex order calculation logic, optimize database queries, and improve the overall structure of the order management code.
5. Performance Optimization for Mobile Apps
Mobile applications often require careful optimization due to device limitations. The refactoring tool can help identify and resolve performance bottlenecks in mobile app code.
Example: A popular mobile game written in Swift for iOS devices could benefit from the tool’s optimization capabilities. It might suggest more efficient algorithms for game physics calculations, optimize memory usage in rendering routines, or improve battery life by refactoring background processes.
6. Codebase Standardization in Team Environments
In large development teams, maintaining consistent coding standards can be challenging. The refactoring tool can help enforce these standards across the entire codebase.
Example: A multinational corporation with development teams spread across different countries could use the tool to ensure that all Java code adheres to the same style guidelines, naming conventions, and best practices, regardless of which team wrote it.
Frequently Asked Questions (FAQ)
Q1: Can the Code Refactoring Tool handle multiple programming languages?
A1: Yes, our tool is designed to work with a wide range of programming languages, including but not limited to Java, Python, JavaScript, C++, and Ruby. When using the tool, make sure to specify the correct language in the designated field for optimal results.
Q2: How does the tool ensure that the refactored code maintains the original functionality?
A2: The tool uses advanced algorithms to analyze the code’s structure and behavior before making any changes. It follows the principle of behavior preservation, which means that while the internal structure of the code may change, its external functionality remains the same. However, it’s always a good practice to thoroughly test the refactored code to ensure no unintended changes have been introduced.
Q3: Can I customize the refactoring rules or patterns used by the tool?
A3: Currently, the tool uses a set of predefined refactoring patterns based on industry best practices. While direct customization is not available, you can influence the refactoring process by specifying your concerns or focus areas in the optional field provided.
Q4: Is the tool suitable for refactoring large codebases?
A4: The tool is designed to handle code snippets and individual functions or classes. For large codebases, we recommend breaking down the refactoring process into smaller, manageable parts. This approach allows for more focused refactoring and easier verification of the results.
Q5: How often should I use the Code Refactoring Tool?
A5: The frequency of refactoring depends on your development cycle and project needs. Many developers find it beneficial to refactor code regularly, such as before adding new features, during code reviews, or as part of routine maintenance. Consistent, smaller refactoring efforts often lead to better long-term code health than infrequent, large-scale refactoring projects.
Q6: Can the tool help in learning better coding practices?
A6: Absolutely! The Code Refactoring Tool can be an excellent learning resource, especially for junior developers. By analyzing the changes suggested by the tool, you can gain insights into efficient coding patterns, best practices, and language-specific optimizations. It’s like having an experienced mentor review and improve your code.
Q7: Does the tool support refactoring of object-oriented code?
A7: Yes, the tool is capable of refactoring object-oriented code. It can suggest improvements related to class structures, inheritance hierarchies, and the application of design patterns. This makes it particularly useful for languages like Java, C++, and Python when used in an object-oriented context.
Q8: How does the tool handle comments and documentation in the code?
A8: The tool preserves existing comments and documentation while refactoring. It recognizes the importance of well-documented code and aims to maintain or enhance the clarity provided by comments. In some cases, it might suggest improvements to the documentation to better reflect the refactored code structure.
Q9: Can the tool help in preparing code for cloud deployment?
A9: While the tool doesn’t specifically target cloud deployment, many of its refactoring suggestions can indirectly benefit cloud-ready code. For example, improving code efficiency and reducing complexity can lead to better performance and scalability in cloud environments. However, for cloud-specific optimizations, you might need to combine the tool’s output with cloud-specific best practices.
Q10: Is it possible to undo the changes suggested by the tool?
A10: The Code Refactoring Tool doesn’t directly modify your original code. It provides suggestions in a separate output area. This means you have full control over which changes to implement. We recommend using version control systems like Git when applying the suggested changes to your actual codebase, allowing you to easily revert if needed.
By leveraging our Code Refactoring Tool effectively, you can significantly improve your code quality, learn better coding practices, and enhance the overall efficiency of your software development process. Remember, while the tool provides valuable suggestions, the final decision on implementing changes should always involve careful consideration and testing to ensure the best outcomes for your specific project needs.
Important Disclaimer
The calculations, results, and content provided by our tools are not guaranteed to be accurate, complete, or reliable. Users are responsible for verifying and interpreting the results. Our content and tools may contain errors, biases, or inconsistencies. We reserve the right to save inputs and outputs from our tools for the purposes of error debugging, bias identification, and performance improvement. External companies providing AI models used in our tools may also save and process data in accordance with their own policies. By using our tools, you consent to this data collection and processing. We reserve the right to limit the usage of our tools based on current usability factors. By using our tools, you acknowledge that you have read, understood, and agreed to this disclaimer. You accept the inherent risks and limitations associated with the use of our tools and services.
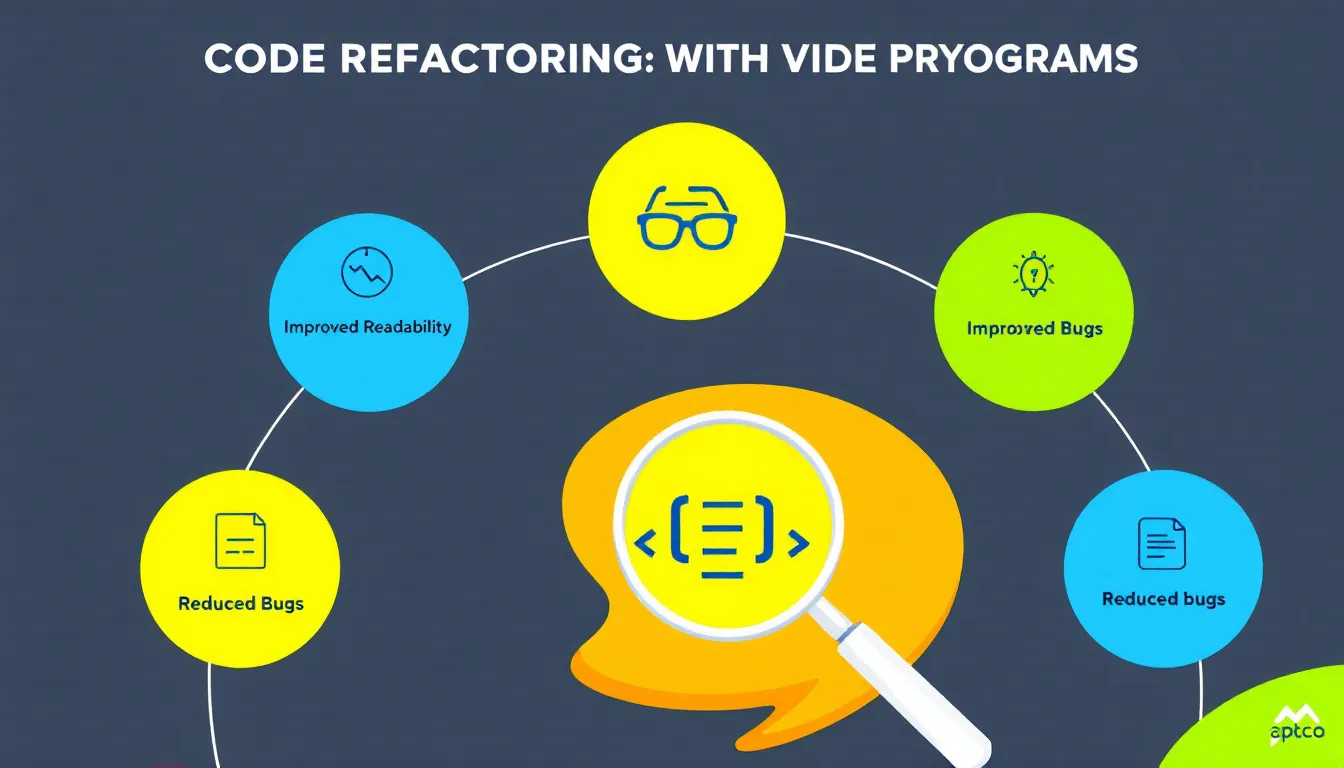