JavaScript Logging Statement Remover
Processing...
Is this tool helpful?
How to Use the Logging Statement Removal Tool Effectively
To utilize the Logging Statement Removal Tool efficiently, follow these simple steps:
- Enter your JavaScript code: In the provided text area, paste the JavaScript code that contains logging statements you wish to remove. For example, you could input:
function calculateTotal(items) {
gs.log(“Calculating total for ” + items.length + ” items”);
let total = 0;
for (let item of items) {
gs.debug(“Processing item: ” + item.name);
total += item.price;
}
gs.info(“Total calculated: ” + total);
return total;
}
- Click “Remove Logging Statements”: After entering your code, click the blue “Remove Logging Statements” button. The tool will process your input and remove all logging statements.
- Review the results: The updated JavaScript code will appear in the “Updated JavaScript Code” section below the form. You’ll see your code with all logging statements removed.
- Copy the clean code: Use the “Copy to Clipboard” button to easily copy the updated code for use in your project.
Another example of code you might input could be:
function updateUserProfile(userId, newData) {
gs.logWarning(“Updating user profile for ID: ” + userId);
try {
let user = getUserById(userId);
gs.debug(“Retrieved user data: ” + JSON.stringify(user));
Object.assign(user, newData);
saveUser(user);
gs.info(“User profile updated successfully”);
return true;
} catch (error) {
gs.logError(“Error updating user profile: ” + error.message);
return false;
}
}
By using this tool, you can quickly clean up your code and remove all logging statements, making it production-ready with minimal effort.
Introduction to the Logging Statement Removal Tool
The Logging Statement Removal Tool is an innovative and time-saving solution designed to assist developers in preparing their JavaScript code for production environments. This powerful utility automatically identifies and removes various types of logging statements, including gs.log, gs.info, gs.debug, gs.logError, and gs.logWarning functions, from your code.
Logging statements are invaluable during the development and debugging phases of a project. They provide crucial insights into the code’s execution flow, variable states, and potential issues. However, these same statements can become problematic when left in production code, potentially causing performance bottlenecks, exposing sensitive information, or cluttering server logs with unnecessary data.
The primary purpose of this tool is to streamline the transition from development to production by automating the tedious task of manually removing logging statements. By doing so, it not only saves developers significant time but also reduces the risk of human error in this critical process.
Key Features and Benefits
- Comprehensive Detection: The tool identifies all common logging functions used in ServiceNow development environments.
- Precision Removal: It accurately removes logging statements without affecting surrounding code.
- Time Efficiency: Automates a process that would otherwise require manual code review and editing.
- Error Prevention: Minimizes the risk of accidentally leaving debug information in production code.
- Code Cleanliness: Produces cleaner, more maintainable code by removing unnecessary statements.
- Performance Optimization: Helps improve runtime performance by eliminating logging overhead.
Benefits of Using the Logging Statement Removal Tool
1. Time Savings
One of the most significant advantages of using this tool is the substantial time savings it offers. Manually reviewing and removing logging statements from large codebases can be an extremely time-consuming task. This tool automates the process, allowing developers to clean up their code in seconds rather than hours or even days.
2. Increased Productivity
By automating the removal of logging statements, developers can focus their time and energy on more critical aspects of their projects. This increased productivity can lead to faster development cycles and more efficient use of resources.
3. Improved Code Quality
Removing unnecessary logging statements results in cleaner, more maintainable code. This improved code quality makes it easier for developers to read, understand, and modify the codebase in the future, leading to more efficient long-term maintenance.
4. Enhanced Security
Logging statements often contain sensitive information that should not be exposed in production environments. By systematically removing these statements, the tool helps prevent potential security vulnerabilities and data leaks.
5. Optimized Performance
Logging operations, especially when frequent or verbose, can impact the performance of an application. By removing these statements, the tool helps optimize the code’s runtime performance, potentially leading to faster and more efficient applications.
6. Consistency Across Projects
Using an automated tool ensures a consistent approach to removing logging statements across different projects and team members. This consistency can be particularly valuable in large organizations or open-source projects with multiple contributors.
7. Reduced Cognitive Load
Developers no longer need to mentally keep track of which logging statements to remove or worry about accidentally leaving debug code in production. This reduced cognitive load allows them to focus on more complex and creative aspects of software development.
Addressing User Needs and Solving Specific Problems
The Logging Statement Removal Tool addresses several critical needs and solves specific problems that developers frequently encounter in their workflow:
1. Streamlining the Development to Production Transition
One of the most significant challenges in software development is ensuring a smooth transition from development to production environments. This tool bridges that gap by automating a crucial step in the process. It allows developers to freely use logging statements during development and testing, knowing they can easily remove them before deployment.
2. Eliminating Human Error
Manual removal of logging statements is prone to human error. Developers might accidentally leave some statements behind or inadvertently remove important code. This tool eliminates such risks by systematically identifying and removing only the specified logging functions.
3. Handling Large Codebases
As projects grow in size and complexity, manually managing logging statements becomes increasingly challenging. The tool scales effortlessly, capable of processing thousands of lines of code in seconds, making it invaluable for large-scale applications.
4. Maintaining Code Readability
Excessive logging statements can clutter code and make it harder to read and understand. By removing these statements, the tool helps maintain clean, readable code that focuses on the core functionality.
5. Ensuring Consistent Code Quality
In team environments, different developers may have varying practices regarding logging statements. This tool ensures a consistent approach across the entire codebase, regardless of individual coding styles.
6. Facilitating Code Reviews
Code reviewers often need to mentally filter out logging statements to focus on the actual logic. By removing these statements, the tool makes the code review process more efficient and effective.
Example Usage and Problem Solving
Let’s consider a real-world scenario to illustrate how the Logging Statement Removal Tool solves specific problems:
Suppose we have a function that processes user orders:
function processOrder(orderId) {
gs.log(“Starting to process order: ” + orderId);
let order = getOrderDetails(orderId);
gs.debug(“Order details: ” + JSON.stringify(order));
if (!validateOrder(order)) {
gs.logWarning(“Invalid order detected: ” + orderId);
return false;
}
try {
let result = submitOrderToWarehouse(order);
gs.info(“Order submitted successfully: ” + orderId);
return result;
} catch (error) {
gs.logError(“Error processing order: ” + error.message);
return false;
}
}
This function contains multiple logging statements that are useful during development but should not be present in the production code. Using the Logging Statement Removal Tool, we can quickly clean up this function:
function processOrder(orderId) {
let order = getOrderDetails(orderId);
if (!validateOrder(order)) {
return false;
}
try {
let result = submitOrderToWarehouse(order);
return result;
} catch (error) {
return false;
}
}
The resulting code is cleaner, more focused, and ready for production use. It maintains the core functionality while removing all debugging and logging statements.
Practical Applications and Use Cases
The Logging Statement Removal Tool has a wide range of practical applications across various development scenarios. Here are some key use cases:
1. Pre-Deployment Code Cleanup
Before deploying an application to a production environment, developers can use this tool as part of their pre-deployment checklist. It ensures that no debugging or logging statements make their way into the live environment, maintaining optimal performance and security.
2. Code Auditing and Compliance
In industries with strict compliance requirements, such as finance or healthcare, this tool can be an essential part of the code auditing process. It helps ensure that no potentially sensitive information is being logged in production environments, aiding in compliance with regulations like GDPR or HIPAA.
3. Open Source Project Maintenance
Maintainers of open-source projects can use this tool to clean up contributions from various developers before merging them into the main codebase. This ensures a consistent code style and prevents the accumulation of unnecessary logging statements.
4. Legacy Code Modernization
When working with legacy codebases that may have accumulated numerous logging statements over time, this tool can be invaluable in cleaning up and modernizing the code. It allows developers to quickly remove outdated debugging code without the risk of manually introducing errors.
5. Continuous Integration Pipelines
The tool can be integrated into continuous integration (CI) pipelines to automatically remove logging statements as part of the build process. This ensures that even if developers forget to remove logging statements, they won’t make it to the final build.
6. Performance Optimization
In performance-critical applications, removing logging statements can provide a noticeable improvement in execution speed. Developers can use this tool as part of their optimization process to eliminate any performance overhead from logging operations.
7. Code Review Assistance
During code reviews, reviewers can use this tool to generate a “clean” version of the code, making it easier to focus on the core logic and functionality without the distraction of logging statements.
Example Use Case: Preparing a Web Application for Production
Let’s consider a practical example of preparing a web application for production deployment:
A team has developed a new e-commerce platform with extensive logging throughout the codebase for debugging purposes. Before launching, they need to ensure all these logging statements are removed. The application includes various modules such as user authentication, product catalog, shopping cart, and order processing.
Without the Logging Statement Removal Tool, the team would need to manually review thousands of lines of code across multiple files, a process that could take days and be prone to errors. With the tool, they can:
- Run the tool on each module’s code, quickly removing all logging statements.
- Verify the cleaned code to ensure core functionality remains intact.
- Integrate the tool into their build process to automatically clean any new code additions.
This process significantly reduces the preparation time for production deployment, minimizes the risk of exposing sensitive information through logs, and ensures a consistent, clean codebase across all modules.
Frequently Asked Questions (FAQ)
Q1: What types of logging statements does this tool remove?
A1: The tool is designed to remove logging statements that use the gs.log, gs.info, gs.debug, gs.logError, and gs.logWarning functions, which are commonly used in ServiceNow development environments.
Q2: Can I use this tool with JavaScript frameworks other than ServiceNow?
A2: While the tool is optimized for ServiceNow logging functions, it can be adapted to work with other JavaScript logging patterns. However, you may need to modify the tool’s configuration to recognize different logging function names.
Q3: Will removing logging statements affect my code’s functionality?
A3: No, the tool is designed to remove only logging statements without affecting the core functionality of your code. However, it’s always a good practice to test your code thoroughly after making any changes.
Q4: How fast is the process of removing logging statements?
A4: The tool processes code very quickly, typically in a matter of seconds, even for large files. The exact speed depends on the size and complexity of your code.
Q5: Can I see what changes the tool has made to my code?
A5: Yes, the tool provides the updated JavaScript code in the results section, allowing you to review all changes made. You can compare this with your original code to see exactly what was removed.
Q6: Is it possible to keep some logging statements while removing others?
A6: The current version of the tool removes all recognized logging statements. If you need to retain certain logs, you might need to manually add them back or use a different logging method that the tool doesn’t recognize.
Q7: Can this tool be integrated into my development workflow or IDE?
A7: While the tool is currently a standalone web-based utility, it’s possible to integrate its functionality into various development workflows or IDEs through custom scripts or plugins.
Q8: What should I do if I accidentally remove important code?
A8: The tool is designed to only remove logging statements, not functional code. However, it’s always a good practice to work with version-controlled code and create backups before making significant changes.
Q9: How often should I use this tool in my development process?
A9: It’s recommended to use the tool as part of your pre-production or deployment process. You can also use it periodically during development to clean up your working code, especially before code reviews or merges.
Q10: Can this tool handle minified or obfuscated JavaScript code?
A10: The tool works best with readable, non-minified JavaScript code. Minified or obfuscated code may pose challenges in accurately identifying logging statements.
Important Disclaimer
The calculations, results, and content provided by our tools are not guaranteed to be accurate, complete, or reliable. Users are responsible for verifying and interpreting the results. Our content and tools may contain errors, biases, or inconsistencies. We reserve the right to save inputs and outputs from our tools for the purposes of error debugging, bias identification, and performance improvement. External companies providing AI models used in our tools may also save and process data in accordance with their own policies. By using our tools, you consent to this data collection and processing. We reserve the right to limit the usage of our tools based on current usability factors. By using our tools, you acknowledge that you have read, understood, and agreed to this disclaimer. You accept the inherent risks and limitations associated with the use of our tools and services.
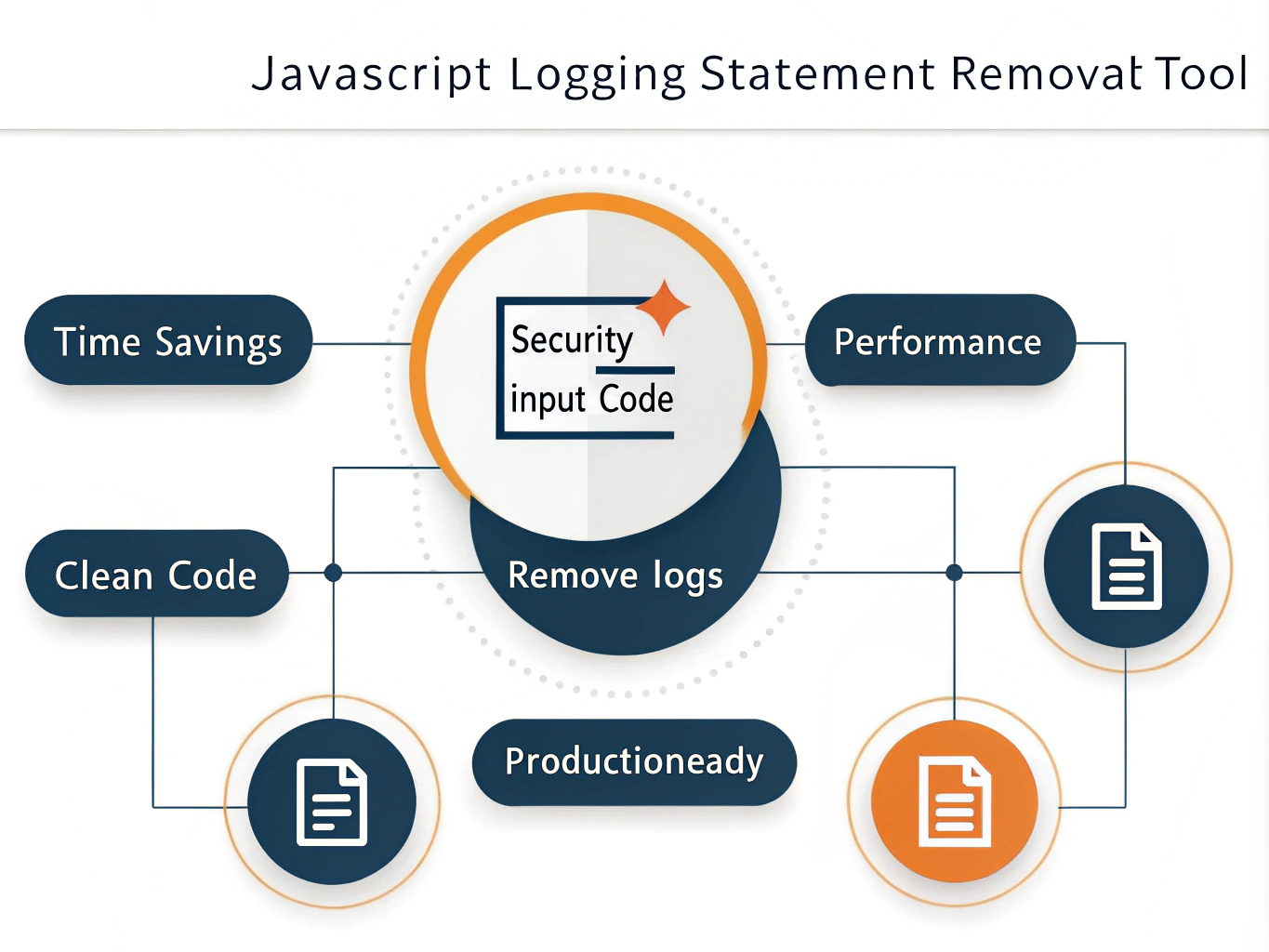