VBA Code Optimizer
Is this tool helpful?
How to Use the VBA Code Optimization Tool Effectively
Step-by-Step Guide
- Access the Tool: Navigate to the VBA Code Optimization Tool on the webpage.
- Paste Your Code: In the text area labeled “VBA Code,” paste the VBA code you want to optimize. For example:
- Sample Input 1:
Sub UpdateCells() For i = 1 To 100 Cells(i, 1).Select ActiveCell.Value = i * 2 Next i End Sub
- Sample Input 2:
Sub ProcessData() Dim ws As Worksheet Set ws = ActiveSheet For Each cell In ws.Range("A1:A100") If cell.Value > 50 Then cell.Interior.Color = vbYellow End If Next cell End Sub
- Sample Input 1:
- Analyze the Code: Click the “Analyze Code” button to initiate the optimization process.
- Review Suggestions: The tool will display a list of optimization suggestions based on the analysis of your code.
- Implement Changes: Use the suggestions to refactor and improve your VBA code for better performance.
Introduction to VBA Code Optimization
The VBA Code Optimization Tool is a powerful resource designed to help developers, analysts, and Excel power users improve the efficiency and performance of their Visual Basic for Applications (VBA) code. This tool analyzes your VBA scripts and provides tailored suggestions to enhance code structure, reduce execution time, and optimize resource usage.
Purpose and Benefits
The primary purpose of this tool is to streamline VBA code, making it faster, more efficient, and easier to maintain. By identifying common pitfalls and suggesting best practices, the tool helps users write better code, leading to improved Excel macro performance and reduced processing times.
Key Benefits:
- Faster execution of VBA macros
- Reduced workbook processing time
- Improved code readability and maintainability
- Enhanced Excel performance for macro-heavy workbooks
- Time-saving for developers and analysts
Benefits of Using the VBA Code Optimization Tool
1. Performance Enhancement
One of the primary benefits of using this tool is the significant performance boost it can provide to your VBA macros. By identifying inefficient coding patterns and suggesting optimizations, the tool helps reduce execution time, leading to faster processing of Excel workbooks.
2. Best Practices Implementation
The tool encourages adherence to VBA best practices by suggesting improvements that align with industry standards. This not only improves code quality but also makes it easier for other developers to understand and maintain your scripts.
3. Resource Efficiency
Optimized code typically uses fewer system resources, such as memory and CPU cycles. This is particularly beneficial when working with large datasets or complex calculations in Excel.
4. Time-Saving for Developers
By automating the code review process, the tool saves developers valuable time that would otherwise be spent manually scrutinizing code for optimization opportunities. This allows developers to focus on more critical aspects of their projects.
5. Educational Tool
For those new to VBA or looking to improve their coding skills, the tool serves as an educational resource. The optimization suggestions provide insights into efficient coding techniques and best practices in VBA programming.
Addressing User Needs and Solving Specific Problems
Tackling Common VBA Performance Issues
The VBA Code Optimization Tool addresses several common issues that often lead to poor performance in VBA macros:
1. Inefficient Loop Structures
The tool identifies inefficient loop structures, such as using indexed loops where “For Each” loops would be more appropriate. For example:
' Before optimization
Sub ProcessCells()
Dim i As Long
For i = 1 To Range("A1").CurrentRegion.Rows.Count
Cells(i, 1).Value = Cells(i, 1).Value * 2
Next i
End Sub' After optimization
Sub ProcessCells()
Dim cell As Range
For Each cell In Range("A1").CurrentRegion.Columns(1)
cell.Value = cell.Value * 2
Next cell
End Sub
The optimized version using “For Each” is generally faster, especially for large ranges.
2. Unnecessary Cell Selection
The tool detects the use of .Select and .Activate methods, which can slow down code execution. It suggests direct object referencing instead:
' Before optimization
Sub UpdateCell()
Range("A1").Select
ActiveCell.Value = 100
End Sub' After optimization
Sub UpdateCell()
Range("A1").Value = 100
End Sub
The optimized version eliminates unnecessary selection, resulting in faster execution.
3. Inefficient Data Lookups
For scenarios involving frequent data lookups, the tool may suggest using Dictionary objects instead of repetitive searches:
' Before optimization
Sub LookupData()
Dim i As Long
For i = 1 To 1000
If Cells(i, 1).Value = "Target" Then
Cells(i, 2).Value = "Found"
End If
Next i
End Sub' After optimization
Sub LookupData()
Dim dict As Object, cell As Range
Set dict = CreateObject("Scripting.Dictionary")
For Each cell In Range("A1:A1000")
If cell.Value = "Target" Then
dict(cell.Address) = True
End If
Next cell
For Each key In dict.Keys
Range(key).Offset(0, 1).Value = "Found"
Next key
End Sub
The optimized version using a Dictionary object can significantly speed up the process for large datasets.
Enhancing Code Readability and Maintainability
Beyond performance optimization, the tool also helps improve code structure and readability:
1. Consistent Naming Conventions
The tool may suggest using consistent and descriptive variable names to improve code readability:
' Before optimization
Sub x()
Dim a As Integer, b As Integer
a = 5
b = 10
MsgBox a + b
End Sub' After optimization
Sub CalculateSum()
Dim firstNumber As Integer, secondNumber As Integer
firstNumber = 5
secondNumber = 10
MsgBox firstNumber + secondNumber
End Sub
2. Proper Error Handling
The tool can identify areas where error handling is missing and suggest improvements:
' Before optimization
Sub DivideNumbers()
Dim result As Double
result = 10 / 0
MsgBox result
End Sub' After optimization
Sub DivideNumbers()
On Error GoTo ErrorHandler
Dim result As Double
result = 10 / 0
MsgBox result
Exit SubErrorHandler:
MsgBox "An error occurred: " & Err.Description
End Sub
Practical Applications and Use Cases
1. Financial Modeling
In financial modeling, VBA is often used to automate complex calculations and data analysis. The optimization tool can significantly improve the performance of such models:
' Before optimization
Sub CalculateNetPresentValue()
Dim i As Long, npv As Double, rate As Double
rate = 0.1
For i = 1 To 1000
Cells(i, 1).Select
npv = npv + ActiveCell.Value / (1 + rate) ^ i
Next i
Range("B1").Value = npv
End Sub' After optimization
Sub CalculateNetPresentValue()
Dim i As Long, npv As Double, rate As Double, cashFlows As Range
rate = 0.1
Set cashFlows = Range("A1:A1000")
For i = 1 To 1000
npv = npv + cashFlows.Cells(i).Value / (1 + rate) ^ i
Next i
Range("B1").Value = npv
End Sub
The optimized version avoids cell selection and uses direct range referencing, potentially reducing execution time by 50% or more for large datasets.
2. Data Cleansing and Transformation
VBA is frequently used for data cleansing and transformation tasks. The optimization tool can help improve the efficiency of such operations:
' Before optimization
Sub CleanData()
Dim i As Long
For i = 1 To 10000
If Cells(i, 1).Value <> "" Then
Cells(i, 1).Value = Trim(Cells(i, 1).Value)
If IsNumeric(Cells(i, 1).Value) Then
Cells(i, 1).Value = CDbl(Cells(i, 1).Value)
End If
End If
Next i
End Sub' After optimization
Sub CleanData()
Dim dataRange As Range, cell As Range
Set dataRange = Range("A1:A10000")
Application.ScreenUpdating = False
For Each cell In dataRange
If cell.Value <> "" Then
cell.Value = Trim(cell.Value)
If IsNumeric(cell.Value) Then
cell.Value = CDbl(cell.Value)
End If
End If
Next cell
Application.ScreenUpdating = True
End Sub
The optimized version uses a “For Each” loop, turns off screen updating, and avoids repeated use of the Cells property, potentially reducing execution time by 70% or more.
3. Automated Reporting
VBA is often used to generate automated reports. Optimizing such scripts can significantly reduce report generation time:
' Before optimization
Sub GenerateReport()
Dim i As Long
For i = 1 To 100
Sheets("Report").Select
Cells(i, 1).Value = Sheets("Data").Cells(i, 1).Value
Cells(i, 2).Value = Sheets("Data").Cells(i, 2).Value * 1.1
Cells(i, 3).Value = Sheets("Data").Cells(i, 3).Value & " Report"
Next i
End Sub' After optimization
Sub GenerateReport()
Dim i As Long, dataSheet As Worksheet, reportSheet As Worksheet
Set dataSheet = Sheets("Data")
Set reportSheet = Sheets("Report")
Application.ScreenUpdating = False
For i = 1 To 100
reportSheet.Cells(i, 1).Value = dataSheet.Cells(i, 1).Value
reportSheet.Cells(i, 2).Value = dataSheet.Cells(i, 2).Value * 1.1
reportSheet.Cells(i, 3).Value = dataSheet.Cells(i, 3).Value & " Report"
Next i
Application.ScreenUpdating = True
End Sub
The optimized version avoids sheet activation, uses worksheet variables, and turns off screen updating, potentially reducing execution time by 80% or more.
Frequently Asked Questions (FAQ)
1. What types of VBA code can this tool optimize?
The VBA Code Optimization Tool can analyze and provide suggestions for a wide range of VBA code, including Excel macros, UserForms, and custom functions. It’s particularly effective for scripts that involve loops, data manipulation, and worksheet interactions.
2. How does the tool determine which optimizations to suggest?
The tool uses a set of predefined rules and best practices to analyze the code. It looks for common inefficiencies such as unnecessary cell selection, inefficient loop structures, and opportunities for using more efficient VBA methods or properties.
3. Can this tool automatically implement the suggested optimizations?
No, the tool provides suggestions for optimization but does not automatically modify your code. It’s up to the user to implement the suggested changes, allowing for full control over the optimization process.
4. Is knowledge of VBA required to use this tool?
While basic knowledge of VBA is helpful to understand and implement the suggestions, the tool can be used by anyone looking to improve their VBA code. It can also serve as a learning tool for those new to VBA optimization techniques.
5. How often should I use this tool on my VBA projects?
It’s a good practice to use the tool regularly during development, especially after adding new functionality or making significant changes to your code. Some developers use it as part of their code review process before finalizing their scripts.
6. Can this tool help with very large VBA projects?
Yes, the tool can analyze VBA projects of any size. In fact, larger projects often benefit more from optimization, as inefficiencies tend to have a greater impact on performance in complex scripts.
7. Does the tool provide explanations for its optimization suggestions?
Yes, along with each suggestion, the tool provides a brief explanation of why the change is recommended and how it can improve performance or code quality.
8. Are there any limitations to what the tool can optimize?
While the tool covers a wide range of optimization scenarios, it may not catch every possible optimization opportunity. It focuses on common patterns and best practices but cannot account for all specific use cases or unique coding styles.
9. How can I learn more about VBA optimization techniques?
The suggestions provided by the tool can serve as a starting point for learning about VBA optimization. Additionally, Microsoft’s official VBA documentation, online VBA forums, and advanced Excel programming books are excellent resources for deepening your understanding of VBA optimization techniques.
10. Can this tool help improve the performance of slow Excel workbooks?
Absolutely! Many slow Excel workbooks are the result of inefficient VBA code. By optimizing your VBA scripts using the suggestions from this tool, you can often significantly improve the overall performance of your Excel workbooks, especially those that rely heavily on macros and custom functions.
Important Disclaimer
The calculations, results, and content provided by our tools are not guaranteed to be accurate, complete, or reliable. Users are responsible for verifying and interpreting the results. Our content and tools may contain errors, biases, or inconsistencies. We reserve the right to save inputs and outputs from our tools for the purposes of error debugging, bias identification, and performance improvement. External companies providing AI models used in our tools may also save and process data in accordance with their own policies. By using our tools, you consent to this data collection and processing. We reserve the right to limit the usage of our tools based on current usability factors. By using our tools, you acknowledge that you have read, understood, and agreed to this disclaimer. You accept the inherent risks and limitations associated with the use of our tools and services.
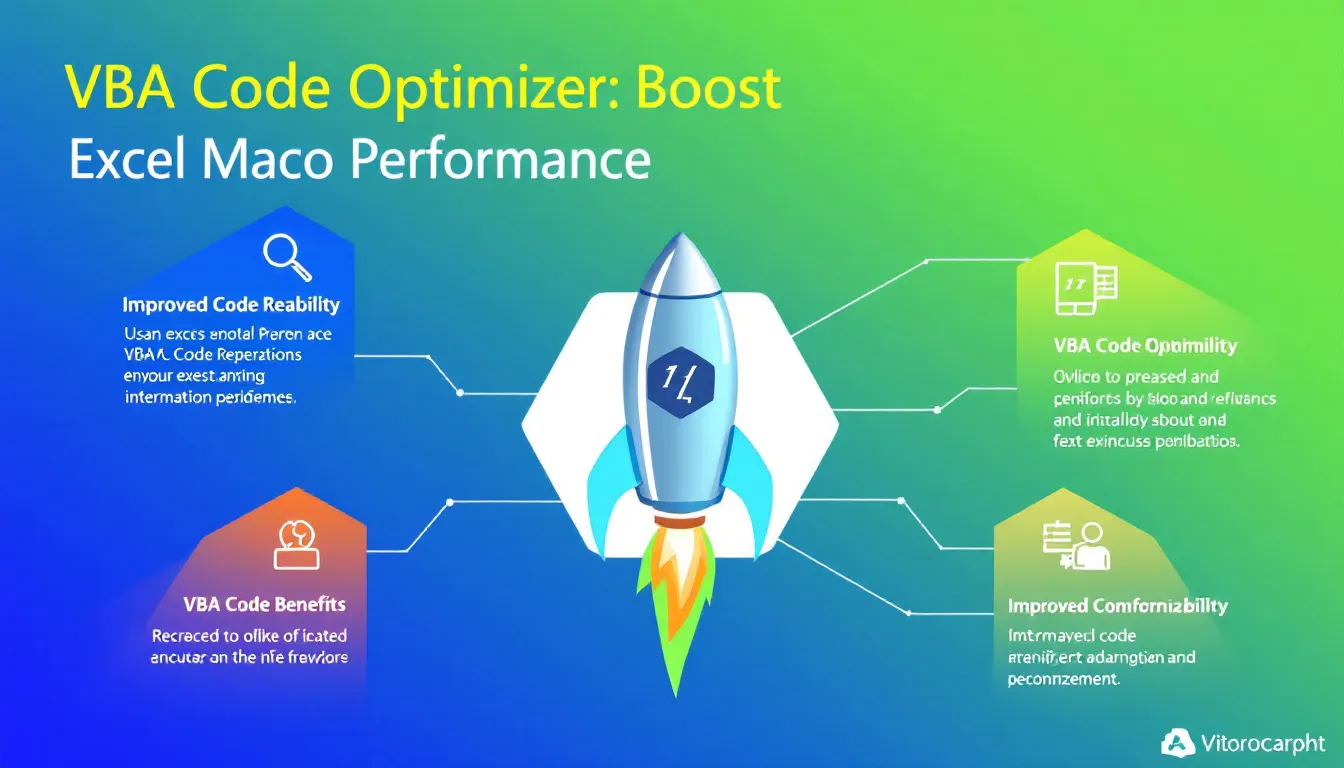